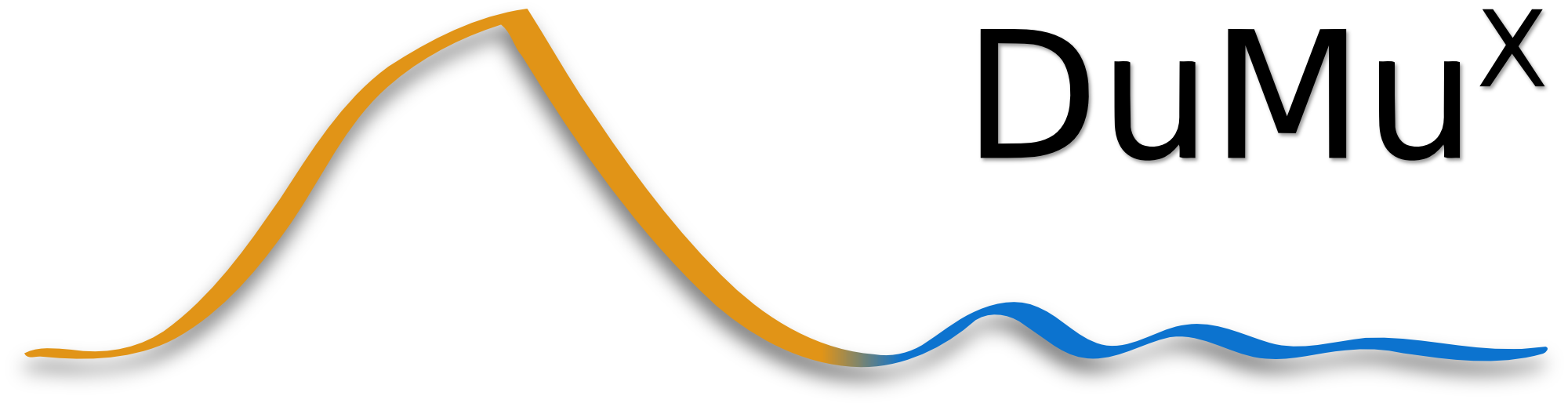
What is DuMuX?
DuMuX is a simulation toolbox mainly aimed at flow and transport processes in porous media. DuMuX is based on the DUNE framework and aims to provide a multitude of numerical models as well as flexible discretization methods for complex non-linear phenomena, such as CO2 sequestration, soil remediation, drug delivery in cancer therapy and more. See our publication for a more detailed description of the goals and motivations behind DuMuX.
Installation
Have a look at the installation guide or use the [DuMuX handbook] 4, chapter 2.
License
DuMuX is licensed under the terms and conditions of the GNU General
Public License (GPL) version 2 or - at your option - any later
version. The GPL can be read online or in the LICENSE.md
file
provided in the topmost directory of the DuMuX source code tree.
Please note that DuMuX' license, unlike DUNE's, does not feature a template exception to the GNU General Public License. This means that you must publish any source code which uses any of the DuMuX header files if you want to redistribute your program to third parties. If this is unacceptable to you, please contact us for a commercial license.
See the file LICENSE.md
for full copying permissions.
Automated Testing
DuMuX features many tests (some unit tests and test problems) that can be run manually. We have experimental support for automated testing with buildbot. Click here (buildbot) to see the latest builds (clicking on a build number will show a detailed overview of the build).